Python math ceil function is one of many functions provided by Python’s math
module. In this article, we’ll see how to use it through examples.
Other similar functions include the floor()
function I wrote about in this article and the round()
function.
Python math ceil function explained
The ceil()
function calculates the smallest integer greater than or equal to the number x
. In other words, it rounds the number x
up to the nearest integer value as shown in the figure below.
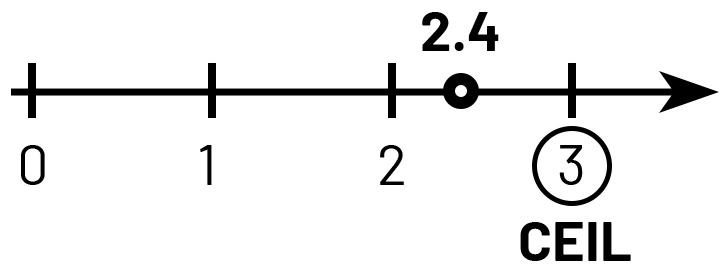
The syntax is as follows:
math.ceil(x)
where x
is the value you want to apply ceiling to.
Below you can find the example of the ceil()
function usage.
>>> import math >>> math.ceil(3.14) 4
To apply the ceiling operation on a particular number or mathematical expression, first import Python’s math
module. Then pass the number to the ceil()
function as the first argument. As a result, you’ll get the nearest integer value greater than or equal to the passed number.
NOTE - Python 2 returns a ceil() function result as a floating point value (float). Python 3 returns it as an integer value (int).
You can see a few examples of using the Python ceil()
method below.
x | math.ceil(x) |
---|---|
3.14 | 4 |
4 | 4 |
2.99999999 | 3 |
-3.14 | -3 |
-4 | -4 |
-2.99999999 | -2 |
math.inf | OverflowError |
Notice that the OverflowError
was raised when math.inf
(Infinity) value was passed to the ceil()
function. This happened because the math.inf
(float value) was attempted to convert to an int value which caused an overflow.
Ceil division in Python
Unlike the floor division that is supported by a special //
operator, there’s no special operator for a ceil division. But there are two ways how you can achieve the same result.
- Define your own function that uses the floor division operator.
def ceil(a, b): return -1 * (-a // b) ceil(7,4) # 2
- Use the Python ceil function directly.
import math math.ceil(7/4) # 2
Summary
Python ceil function is one of the standard functions coming from the math
module. Ceil function returns the smallest integer value greater than or equal to the passed argument. You can use it by importing the math
module. Then simply pass the argument to it and you’ll get ceiled value as a result. To learn more visit Python’s official documentation.
If you want to know how the Python floor()
function works, you can read it in this ZeroToByte article.